In this tutorial, we will walk you through the process of rendering a partial view as a Bootstrap modal in a .NET MVC application. This approach allows you to display additional information or details in a pop-up modal, improving the user experience and keeping the main page clutter-free. We will utilize the power of ASP.NET MVC, Bootstrap, and AJAX to achieve this functionality seamlessly.
Prerequisites
Before we begin, ensure you have the following prerequisites in place:
- Basic knowledge of ASP.NET MVC and Bootstrap.
- Visual Studio installed on your machine.
- Familiarity with C# and Razor syntax.
Setting up the Project
Launch Visual Studio and create a new ASP.NET MVC project.
Install Bootstrap via the NuGet package manager. Open the Package Manager Console and run the following command:
Install-Package bootstrap
- Define the classes for Employee and EmployeeDetail in your model. These classes will represent the data structure for our tutorial.
// Employee class
public class Employee
{
public int Id { get; set; }
public string Name { get; set; }
public EmployeeDetail EmployeeDetail { get; set; }
}
// EmployeeDetail class
public class EmployeeDetail
{
public int Age { get; set; }
public string Email { get; set; }
public int Experience { get; set; }
}
Creating the View
- Add a new view to display the list of employees. In your "Views" folder, create a new file named "Index.cshtml" and add the following content:
@model List<Employee>
<h2>Employee List</h2>
@foreach (var employee in Model)
{
<p>@employee.Name</p>
<button class="btn btn-primary" onclick="openModal(@employee.Id)">View Details</button>
}
<!-- Bootstrap Modal -->
<div class="modal fade" id="employeeModal" tabindex="-1" role="dialog" aria-labelledby="employeeModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="employeeModalLabel">Employee Details</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body" id="modalBody">
<!-- The partial view content will be inserted here -->
</div>
</div>
</div>
</div>
@section Scripts {
<script>
function openModal(employeeId) {
$.ajax({
url: '/Employee/GetEmployeeDetail',
type: 'GET',
data: { id: employeeId },
success: function (data) {
$('#modalBody').html(data);
$('#employeeModal').modal('show');
},
error: function () {
alert('Error occurred while fetching data.');
}
});
}
</script>
}
Creating the Controller
- Add an "EmployeeController" to your project. Right-click on the "Controllers" folder, select "Add," and choose "Controller." Name it "EmployeeController.cs" and add the following code:
public class EmployeeController : Controller
{
// Replace this method with your actual data retrieval logic
private List<Employee> GetSampleEmployeeData()
{
return new List<Employee>
{
new Employee { Id = 1, Name = "John Doe", EmployeeDetail = new EmployeeDetail { Age = 30, Email = "[email protected]", Experience = 5 } },
new Employee { Id = 2, Name = "Jane Smith", EmployeeDetail = new EmployeeDetail { Age = 28, Email = "[email protected]", Experience = 4 } }
};
}
public ActionResult Index()
{
var employees = GetSampleEmployeeData();
return View(employees);
}
// Action to return the partial view with EmployeeDetail data
public PartialViewResult GetEmployeeDetail(int id)
{
// Replace this with your actual data retrieval logic
//var employee = GetSampleEmployeeData().FirstOrDefault(e => e.Id == id);
var employee = new Employee
{
Id = 1,
Name = "John Doe",
EmployeeDetail = new EmployeeDetail
{
Age = 30,
Email = "[email protected]",
Experience = 5
}
};
return PartialView("_EmployeeDetail", employee);
}
}
Creating the Partial View
- In the "Views/Shared" folder, create a new file named "_EmployeeDetail.cshtml" and add the following content:
@model EmployeeDetail
<div class="employee-details">
<h4>Name: @Model.Name</h4>
<p>Age: @Model.Age</p>
<p>Email: @Model.Email</p>
<p>Experience: @Model.Experience years</p>
</div>
Note:
Remember to add reference to Jquery and Bootstrap JS Files.
Output:
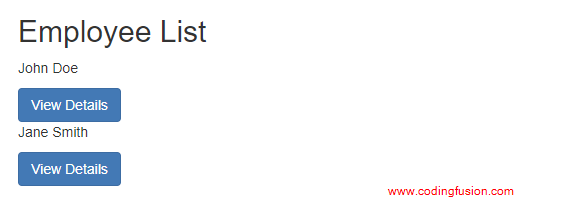
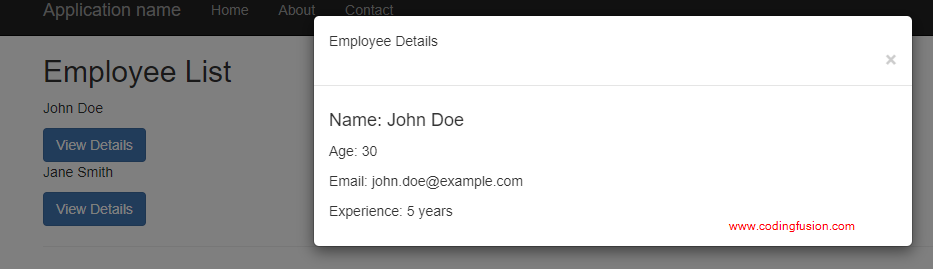
Now your application should be able to display employee details in a Bootstrap modal when you click the "View Details" button for each employee. This approach offers a cleaner and more interactive way of displaying additional information without navigating away from the main page.