Asp .Net tutorial with examples about multiple file upload using FileUpload control in Asp .Net. The asp:FileUpload control provides us a Text Box and browse button which enables users to select a file from our local computer and upload it to the server.
We will aslo discuss some of the most commonly used properties of file upload control like ContentLength,ContentType,FileName and HasFile.
Create FileUpload Control to Upload Multiple Files
This will generate following Output:
Sample code to upload multiple files in asp .net
protected void btnUploadFiles_Click(object sender, EventArgs e)
{
string folderToUploadFiles = Server.MapPath("MyDocuments");
string fileName = string.Empty;
string namesOfUploadedFiles = string.Empty;
if (myFileUpload.HasFiles) //-- Check if user has selected files or not.
{
foreach (HttpPostedFile file in myFileUpload.PostedFiles)
{
//-- Get file name of file to upload.
fileName = file.FileName;
//-- Create folder if it is not already exists
if (!Directory.Exists(folderToUploadFiles))
{
Directory.CreateDirectory(folderToUploadFiles);
}
myFileUpload.SaveAs(Path.Combine(folderToUploadFiles, fileName));
//-- Make a list of uploaded files
namesOfUploadedFiles += fileName + Environment.NewLine;
}
//-- Show list of uploaded files
lblInfoMessages.Text = namesOfUploadedFiles;
}
}
Fileupload example output:
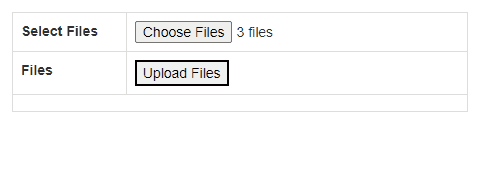
Some of the most used properties during file uploads in Asp .Net
Asp .Net File Upload Control ContentLength
Property
This will Returns the size of the posted file, in bytes. This property is very useful when we want to implement file size validations. Let's say we don't want users to upload files with sizes more than 1 MB.

Asp .Net File Upload Control ContentType
Property
This will Returns the MIME type of the uploaded file. This property is very useful when we want to implement file type validations. Let's say we don't want users to upload PDF or Excel files, Or we only want users to upload Word files.

Asp .Net File Upload Control FileName
Property
This will Returns the full filename. This property is very useful to get the file names of the selected files.
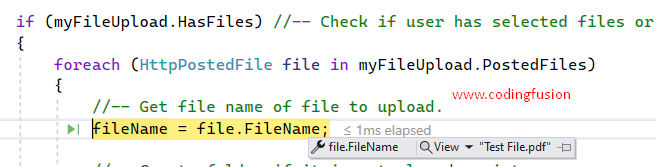
Asp .Net File Upload Control HasFile
Property
This will Specifies whether the control has a file to upload. This property is very useful to check if the user has selected files for upload or not. If there are no files selected we will not execute file upload control to avoid run time errors.
Screenshot:
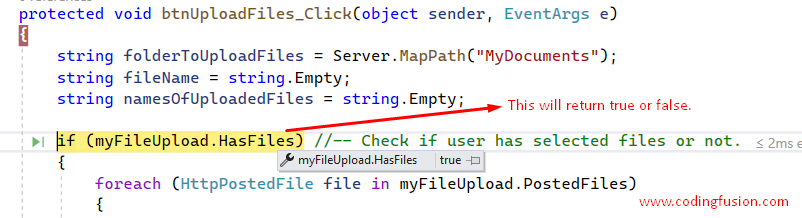