In this
Asp .net MVC tutorial we will learn about
How to create Textbox Autocomplete functionality in asp .net MVC. Jquery autocomplete plugin provides us suggestions when we type in some words in Textbox.
In this asp .net mvc tutorial we will create database, then create MVC controller to get data from database and then we will create view to implement Textbox autocomplete functionality. Our final output will be like this:
For asp .net webforms you can check this:
Textbox autocomplete using jquery in asp .net
Create Database
In this step we will create database table to fetch countries data to create
Jquery AutoComplete TextBox with database In ASP .NET MVC.
CREATE TABLE [dbo].[Country](
[Id] [int] IDENTITY(1,1) NOT NULL PRIMARY KEY,
[Name] [nvarchar](100) NULL,
)
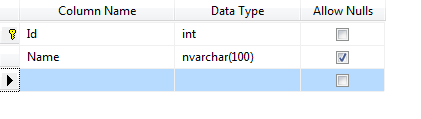
To communicate with database we will use
ADO.NET Entity Data Model
. In this example I have used database first
approach. If you are not familliar with Entity Framework, you can check this tutorial:
Entity Framework Database First, Step by Step Tutorial.
Create Controller
In this step we will create controller and create method to get countries data on request.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace Mvc_JqueryAutoComplete.Controllers
{
public class HomeController : Controller
{
private AutoCompleteEntities db = new AutoCompleteEntities();
public ActionResult Index()
{
return View();
}
[HttpPost]
public JsonResult GetCountries(string Prefix)
{
var Countries = (from c in db.Countries
where c.Name.StartsWith(Prefix)
select new { c.Name, c.Id });
return Json(Countries, JsonRequestBehavior.AllowGet);
}
}
}
Create View
In this step we will create strongly typed view to display
Jquery AutoComplete TextBox with database In ASP .NET MVC. We will also reference required Jquery and CSS files and
call our controller method to get autocomplete data.
@model Mvc_JqueryAutoComplete.Country
@{
ViewBag.Title = "Index";
Layout = "~/Views/Shared/_Layout.cshtml";
}
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-group">
@Html.LabelFor(model => model.Name, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-4">
@Html.TextBoxFor(model => model.Name, htmlAttributes: new { @class = "form-control" })
</div>
</div>
}
<link rel="stylesheet" href="//code.jquery.com/ui/1.11.4/themes/start/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.10.2.js"></script>
<script src="//code.jquery.com/ui/1.11.4/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$("#Name").autocomplete({
source: function (request, response) {
$.ajax({
url: "/Home/GetCountries",
type: "POST",
dataType: "json",
data: { Prefix: request.term },
success: function (data) {
response($.map(data, function (item) {
return { label: item.Name, value: item.Name };
}))
}
})
},
messages: {
noResults: "",
results: function (count) {
return count + (count > 1 ? ' results' : ' result ') + ' found';
}
}
});
})
</script>
Important Notes:
- Textbox Id's must be same.
- Make sure to check
BundleConfig.cs
file inside App_Start
folder to ensure you are not referencing any duplicate CSS or Jquery Scripts.
E.g. If you have already referenced Jquery in your BundleConfig.cs
file, then there is no need to call Jquery script on Index page.
Jquery AutoComplete TextBox with database In ASP .NET MVC Demo