Pass Data From View to Controller using FormCollection in MVC
In this FormCollection example we will learn how to Pass Data From View to Controller using FormCollection in Asp .Net MVC. We will create View using both HTML Controls and HTML Helpers, then we will create controller to get values from view using FormCollection and we will display values using ViewBag.
Create View:
In this step we will create a view and HTML elements. For this tutorial I have used both plain HTML controls and HTML Helper controls.
<body>
<div class="col-sm-4">
@using (Html.BeginForm("CreateEmployee", "Employee"))
{
<table class="table">
<tr>
<td><b>Name</b></td>
<td>
<input class="form-control" name="txtName" placeholder="Enter Name"></td>
</tr>
<tr>
<td><b>Email</b></td>
<td>
@Html.TextBox("txtEmail", null, htmlAttributes: new { @class = "form-control", @placeholder = "Enter Email" })</td>
</tr>
<tr>
<td><b>Age</b></td>
<td>
<input class="form-control" name="txtAge" placeholder="Enter Age"></td>
</tr>
<tr>
<td><b>Gender</b></td>
<td>
<input type="radio" name="gender" checked="checked" value="Male">Male
<input type="radio" name="gender" value="Female">Female
</td>
</tr>
<tr>
<td> </td>
<td>
<input type="submit" value="Submit" class="btn btn-primary" />
</td>
</tr>
</table>
}
@*To Show Output*@
@Html.Raw(ViewBag.employeeValues)
</div>
Output:
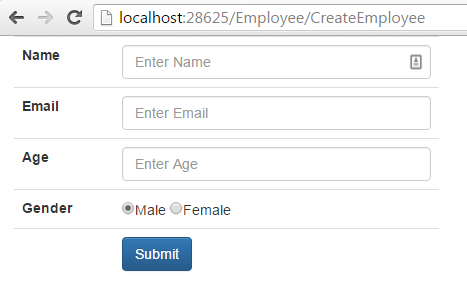
Create Controller:
In this step we will create an EmployeeController
to accept values from view using FormCollection.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Web;
using System.Web.Mvc;
namespace Mvc_Test.Controllers
{
public class EmployeeController : Controller
{
public ActionResult Index()
{
return View();
}
public ActionResult CreateEmployee()
{
return View();
}
[HttpPost]
public ActionResult CreateEmployee(FormCollection frmObj)
{
//--- Getting FormCollection Values.
StringBuilder sbValues = new StringBuilder();
sbValues.Append("Employee Name: " + frmObj["txtName"].ToString() + "
");
sbValues.Append("Employee Email: " + frmObj["txtEmail"].ToString() + "
");
sbValues.Append("Employee Age: " + frmObj["txtAge"].ToString() + "
");
sbValues.Append("Employee Gender: " + frmObj["gender"].ToString());
ViewBag.employeeValues = sbValues.ToString();
return View();
}
}
}
Pass Data From View to Controller using FormCollection in MVC Demo
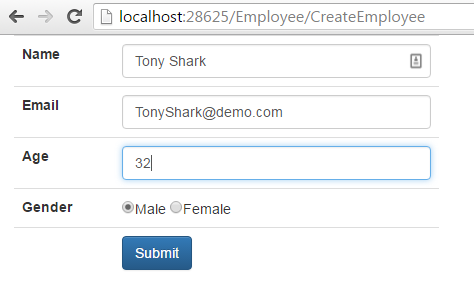
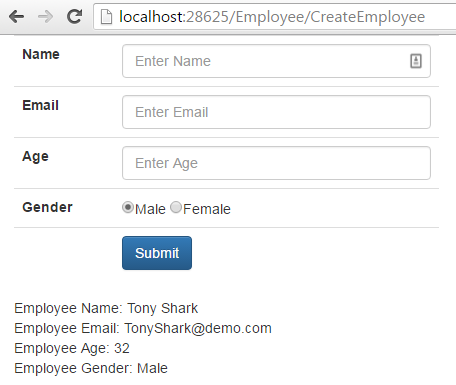