As an ASP.NET MVC developer, you may often encounter scenarios where you need to pass DateTime values as parameters in your URI. Whether it's for filtering data, generating reports, or performing specific actions based on date and time, passing DateTime parameters through the URI can be a common requirement. In this blog post, we'll explore multiple code examples on how to achieve this in a clean and efficient manner.
The Challenge: Encoding DateTime in URIs
HTTP URLs have restrictions on the characters they can contain, and DateTime values can include characters that are not safe to include directly in a URL. Therefore, you need to encode the DateTime values before passing them as URI parameters. There are several ways to achieve this, and we'll cover the most practical and SEO-friendly methods.
ISO 8601 is an international standard for representing dates and times, and it's widely recognized by various programming languages and APIs. The format looks like this: YYYY-MM-DDTHH:mm:ssZ
, where:
YYYY
represents the year (e.g., 2023).
MM
represents the month (01 to 12).
DD
represents the day (01 to 31).
HH
represents the hour (00 to 23).
mm
represents the minute (00 to 59).
ss
represents the second (00 to 59).
Z
indicates that the time is in UTC (optional).
Example:
Passing a DateTime value of August 2nd, 2023, at 10:30 AM would look like this: https://example.com/controller/action?datetime=2023-08-02T10:30:00Z
Controller:
using System;
public ActionResult ActionWithISODateTime()
{
DateTime datetimeValue = DateTime.UtcNow;
string isoDateTime = datetimeValue.ToString( "o" );
return RedirectToAction( "ActionWithDateTimeParam" , new { datetime = isoDateTime });
}
|
View:
<p>The DateTime parameter from the URL is : @Request.QueryString.Get( "datetime" )</p>
<p>Converted To DateTime Value : @DateTime.Parse(Request.QueryString.Get( "datetime" ), null , System.Globalization.DateTimeStyles.RoundtripKind)</p>
|
Output:

2. Use Unix Timestamp
Unix Timestamp is the number of seconds that have elapsed since January 1, 1970 (also known as the "epoch" or "Unix time"). It's a simple and efficient way to represent DateTime values in a numeric format.
Example:
Passing the same DateTime value as a Unix Timestamp would look like this: https://example.com/controller/action?timestamp=1679881800
Controller:
using System;
public class ExampleController : Controller
{
public ActionResult ActionMethod()
{
DateTime datetimeValue = DateTime.UtcNow;
long unixTimestamp = ( long )(datetimeValue - new DateTime(1970, 1, 1)).TotalSeconds;
return RedirectToAction( "Index" , "Employee" , new { datetime = unixTimestamp });
}
}
|
View:
<!-- Access the DateTime parameter in the view -->
<p>The DateTime parameter from the URL is : @Request.QueryString.Get( "datetime" )</p>
<p>Converted To DateTime Value : @DateTimeOffset.FromUnixTimeSeconds(Convert.ToInt64(Request.QueryString.Get( "datetime" ))).UtcDateTime </p>
|
Output:
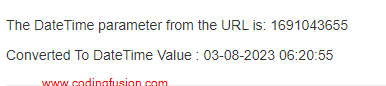
3. URL Encoding
If for some reason, you cannot use the ISO 8601 format or Unix Timestamp, you can also URL-encode the DateTime string. This method will replace special characters with their respective URL-encoded representations, ensuring safe passage through the URI.
Example:
Using URL encoding for the DateTime value August 2nd, 2023, 10:30 AM
would look like this: https://example.com/controller/action?datetime=August%202%2C%202023%2C%2010%3A30%20AM
Controller:
using System;
using System.Web;
public class ExampleController : Controller
{
public ActionResult ActionMethod()
{
DateTime datetimeValue = DateTime.UtcNow;
string encodedDateTime = HttpUtility.UrlEncode(datetimeValue.ToString());
return RedirectToAction( "Index" , "Employee" , new { datetime = encodedDateTime });
}
}
|
View:
<!-- Access the DateTime parameter in the view -->
<p>The DateTime parameter from the URL is : @Request.QueryString.Get( "datetime" )</p>
<p>Converted To DateTime Value : @Html.Raw(HttpUtility.UrlDecode(Request.QueryString.Get( "datetime" )))</p>
|
Output:

Passing DateTime values as URI parameters in ASP.NET MVC is essential for many web applications. By using ISO 8601, Unix Timestamp, or URL encoding, you can efficiently and safely pass DateTime values in your URLs. Remember to use descriptive parameter names and keep your URLs short and clean for better SEO and user experience.