In this article we will learn how to use Google's new Recaptcha "I am not a robot" in asp .net with code behind implementation. reCaptcha is a free service offered by google which helps to fight against bots and spams. Google has launched a new version of reCaptcha which is very secure and good looking from previous versions.
Lets create a simple application in asp .net to implement google's new reCaptcha.
Step1: First of all you need to register your application at google's reCaptcha website. Click on Get reCAPTCHA button.
Step2: Login with your existing account or register for google's account.
Step3: Register a new site.
Note: If you need to test reCaptcha on your localhost. Add localhost in domains section. After Registration you will get Site Key and Secret Key.
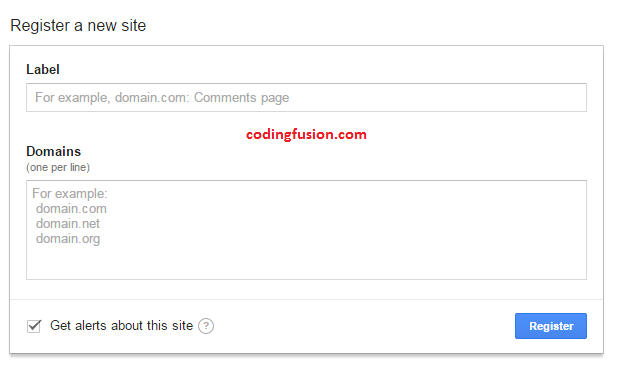
Step4: Add following code into head section website page.
Step5: Add following code in your website page. Here I have placed Recaptcha, Button to implement server side code and Label to show output.
When you submit the form using button ReCaptcha will generate a response in this variable: "g-recaptcha-response". To confirm wether user has verified by the Recaptcha we need to send a GET request to following URL:
https://www.google.com/recaptcha/api/siteverify
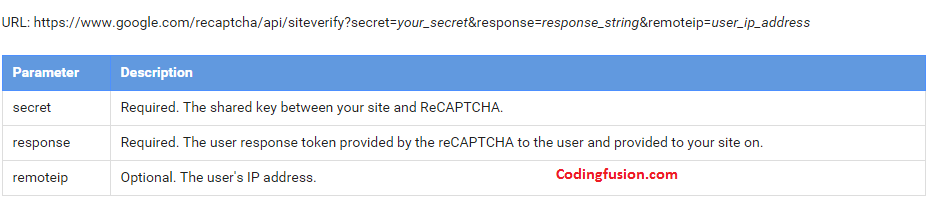
Step5: Server side code.
1) Add following Name spaces:
using System.Runtime.Serialization;
using System.Text;
using System.Net;
using System.Runtime.Serialization.Json;
using System.IO;
2) Create Class to get JSON response.
[DataContract]
public class RecaptchaApiResponse
{
[DataMember(Name = "success")]
public bool Success;
[DataMember(Name = "error-codes")]
public List ErrorCodes; }
You will get response in folowing JSON format.
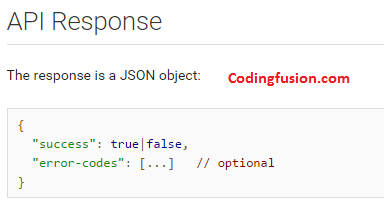
Here is a list of error codes:
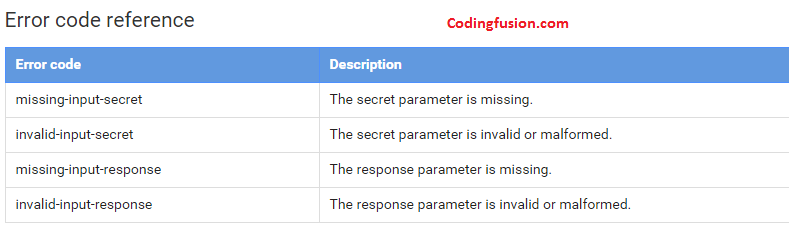
3) Create Method to collect IP Address of the user(Optional).
//--- To get user IP(Optional)
private string GetUserIp()
{
var visitorsIpAddr = string.Empty;
if (Request.ServerVariables["HTTP_X_FORWARDED_FOR"] != null)
{
visitorsIpAddr = Request.ServerVariables["HTTP_X_FORWARDED_FOR"];
}
else if (!string.IsNullOrEmpty(Request.UserHostAddress))
{
visitorsIpAddr = Request.UserHostAddress;
}
return visitorsIpAddr;
}
4) Button Click Event.
protected void btnValidateReCaptcha_Click(object sender, EventArgs e)
{
//start building recaptch api call
var sb = new StringBuilder();
sb.Append("https://www.google.com/recaptcha/api/siteverify?secret=");
//our secret key
var secretKey = "6LeXygATAAAAAKJNnxLEg42upS0LkCwani5g12XV";
sb.Append(secretKey);
//response from recaptch control
sb.Append("&");
sb.Append("response=");
var reCaptchaResponse = Request["g-recaptcha-response"];
sb.Append(reCaptchaResponse);
//client ip address
//---- This Ip address part is optional. If you donot want to send IP address you can
//---- Skip(Remove below 4 lines)
sb.Append("&");
sb.Append("remoteip=");
var clientIpAddress = GetUserIp();
sb.Append(clientIpAddress);
//make the api call and determine validity
using (var client = new WebClient())
{
var uri = sb.ToString();
var json = client.DownloadString(uri);
var serializer = new DataContractJsonSerializer(typeof(RecaptchaApiResponse));
var ms = new MemoryStream(Encoding.Unicode.GetBytes(json));
var result = serializer.ReadObject(ms) as RecaptchaApiResponse;
//--- Check if we are able to call api or not.
if (result == null)
{
lblForMessage.Text = "Captcha was unable to make the api call";
}
else // If Yes
{
//api call contains errors
if (result.ErrorCodes != null)
{
if (result.ErrorCodes.Count > 0)
{
foreach (var error in result.ErrorCodes)
{
lblForMessage.Text = "reCAPTCHA Error: " + error;
}
}
}
else //api does not contain errors
{
if (!result.Success) //captcha was unsuccessful for some reason
{
lblForMessage.Text = "Captcha did not pass, please try again.";
}
else //---- If successfully verified. Do your rest of logic.
{
lblForMessage.Text = "Captcha cleared ";
}
}
}
}
}
Final Output:
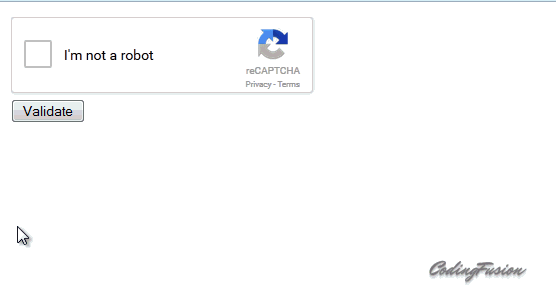